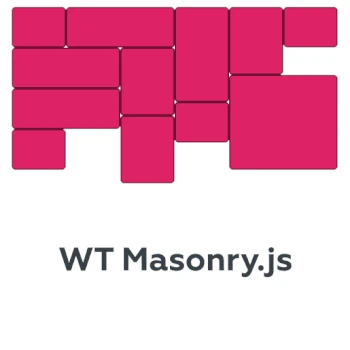
WT Masonry.js
Masonry script that allows you to output images in the form of brickwork. The script is designed in Joomla 4+ WebAsset, which allows you to use it in any extensions of Joomla 4 and Joomla 5. This is a plugin for Joomla developers. It does not provide ready-to-use code.
Description
What is Masonry?
Masonry is a JavaScript grid layout library. It works by placing elements in optimal position based on available vertical space, sort of like a mason fitting stones in a wall. You’ve probably seen it in use all over the Internet.
Enabling an asset in Joomla
<?php
$wa = Factory::getApplication()->getDocument()->getWebAssetManager();
$wa->useScript('wtmasonryjs.local'); // local file
$wa->useScript('wtmasonryjs.remote'); // from CDN
$wa->useScript('imagesloaded.local'); // imagesLoaded.js from local file
$wa->useScript('imagesloaded.remote'); // imagesLoaded.js from CDN
After that, the local or from CDN masonry script will be included in the <head> of the Joomla page.
Also, the imagesLoaded imagesLoaded asset has been added to the plugin, which should be used with a large number of images.
Code example
An example from the official documentation of the script
HTML
<div class="grid">
<div class="grid-item">...</div>
<div class="grid-item grid-item--width2">...</div>
<div class="grid-item">...</div>
...
</div>
CSS
All sizing of items is handled by your CSS.
.grid-item { width: 200px; }
.grid-item--width2 { width: 400px; }
Initialize with jQuery
$('.grid').masonry({
// options
itemSelector: '.grid-item',
columnWidth: 200
});
Initialize with Vanilla JavaScript
var elem = document.querySelector('.grid');
var msnry = new Masonry( elem, {
// options
itemSelector: '.grid-item',
columnWidth: 200
});
// element argument can be a selector string
// for an individual element
var msnry = new Masonry( '.grid', {
// options
});
Connecting imagesLoaded
This script initializes masonry again after loading the next image. This creates a dynamic addition of images to the brickwork.
Initialize with jQuery
// init Masonry
var $grid = $('.grid').masonry({
// options...
});
// layout Masonry after each image loads
$grid.imagesLoaded().progress( function() {
$grid.masonry('layout');
});
Initialize with Vanilla JavaScript
document.addEventListener('DOMContentLoaded',() => {
const masonryGrid = document.getElementById('masonry-test');
var msnry = new Masonry(masonryGrid, {
itemSelector: '.grid-item',
columnWidth: 200,
percentPosition: true
});
imagesLoaded( masonryGrid ).on( 'progress', function() {
// layout Masonry after each image loads
msnry.layout();
});
});
Joomla
- Extension type:
- Plugin
- Folder:
- System
- Joomla version:
- 4.3.4, 5.0.0